Get started with Selenium
Prerequisites
-
A browser is a must. One of Chrome, Firefox, Edge, Safari, Opera, IE, etc.
-
Driver for your browser.
-
geckodriver for Firefox
-
and …
Further more, there are some nice tools such as webdriver-manager, chromedriver-autoinstaller and geckodriver-autoinstaller to check, download and install a suitable driver from python automatically.
Although WSL doesn’t support GUI directly, there are still ways to use WebDrivers/Selenium. Here is a proven method, https://github.com/lackovic/notes/tree/master/Windows/Windows%20Subsystem%20for%20Linux#use-chromedriver-on-wsl. In short, just place ChromeDriver in some system directory in windows for example C:\Windows\System32, and remove .exe extension. After that, your can use from wsl directly.
Common usages
from selenium import webdriver
from selenium.webdriver.common.keys import Keys #provide special keys in the keywords
from webdriver_manager.chrome import ChromeDriverManager
#check ChromeDriver and open a new Chrome browser
browser = webdriver.Chrome(ChromeDriverManager().install())
#load Yahoo homepage
browser.get('http://www.yahoo.com')
assert 'Yahoo' in browser.title
#find the search box
elem = browser.find_element_by_name('p')
#input 'seleniumhq' and type 'ENTER'
elem.send_keys('seleniumhq' + Keys.RETURN)
#elem.send_keys('seleniumhq')
#elem.send_keys(Keys.RETURN)
#close the browser
browser.quit()
ChromeOptions
selenium.webdriver.ChromeOptions()
is equivalent to selenium.webdriver.chrome.options.Options()
.
#open a new ChromeOptions object
options = webdriver.ChromeOptions()
#add agruments
options.add_argument('--headless')
options.add_argument('--disable-gpu')
#open a new Chrome browser
driver = Chrome(options=options)
Further more arguments for Chrome, go after here.
XPath
There is a fantastic blog to guide the usage of XPath. Click here
And you can test a XPath in Chrome Console. F12 –> Console –> Type your XPath like $x(“.//*[@id='id']”)
–> ENTER.
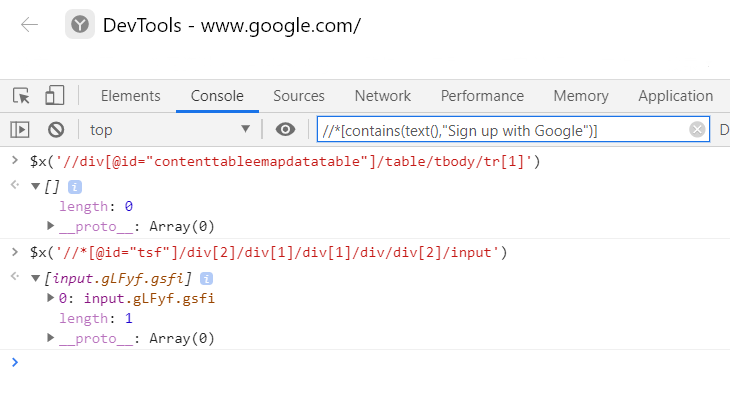
Cases
-
submit the daily report to server, see source code.
-
require cookies from Google Earth Engine, see source code.
Reference
- Selenium
- Selenium - github
- Selenium python API
- List of Chromium Command Line Switches
- XPath in Selenium WebDriver: Complete Tutorial